CSS Basics
Once we have an understanding of how our page is put together, naturally we want to change its appearance. CSS, or Cascading Style Sheets, is the most effective way to do this. From Mozilla's developer docs:
Like HTML, CSS is not a programming language. It's not a markup language either. CSS is a style sheet language. CSS is what you use to selectively style HTML elements.
CSS is composed of rulesets that target "elements" (HTML tags and tag attributes) on our webpages to change their appearance. For instance, the following CSS will make all <p>
tags on the page blue:
p {
color: blue;
}
Linking CSS
You can apply CSS to your webpage in several ways. As inline styles to specific HTML elements, within the <head>
of your HTML document, or via an external stylesheet. For now, let's focus on linking an external stylesheet.
Copy the following code into the head of your personal website index.html
file and save it:
<link href="assets/style.css" rel="stylesheet" />
This will find the style.css
document in your assets
folder. Reload the page and see how it has changed.
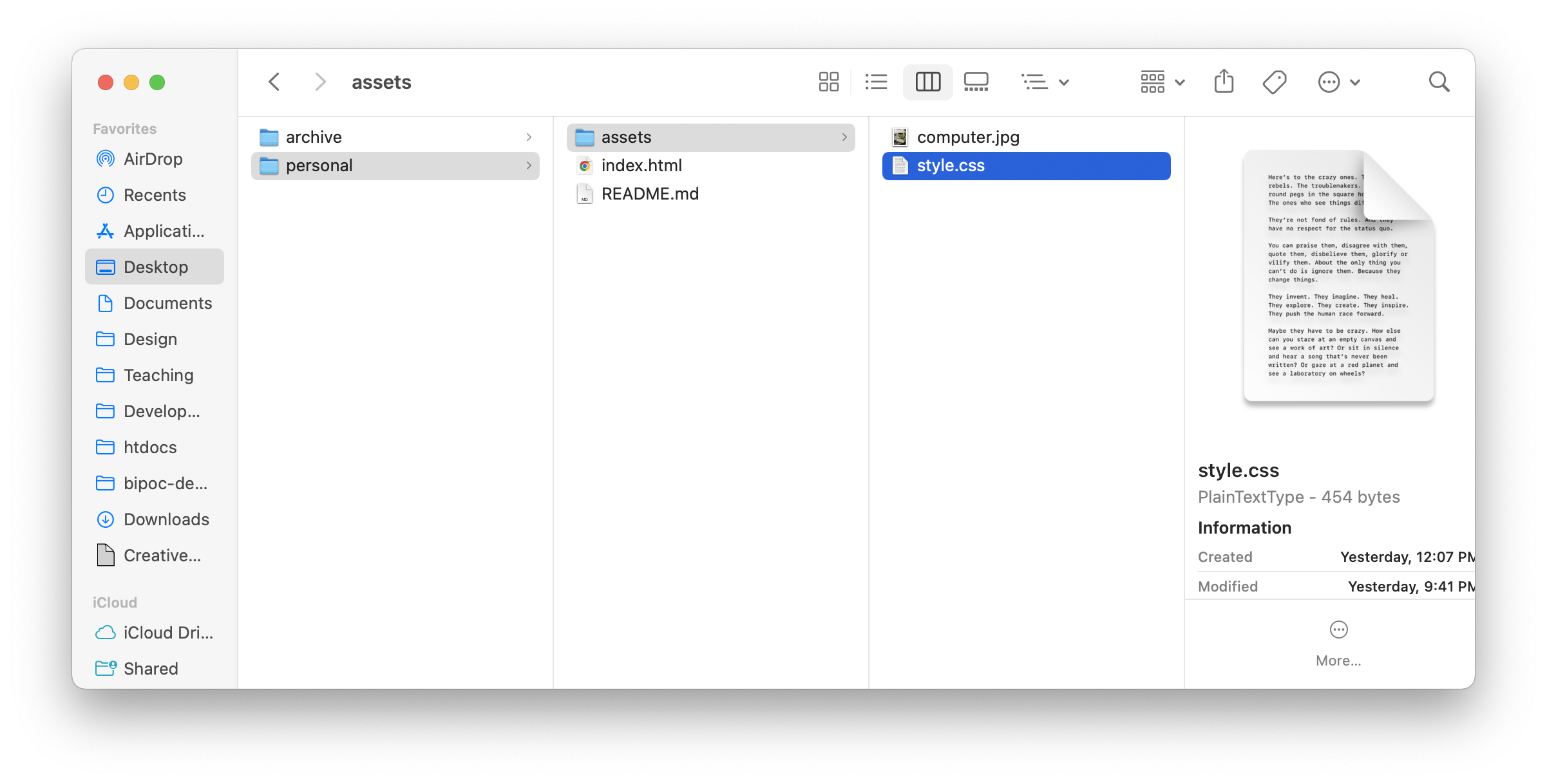
Anatomy of a CSS ruleset

CSS follows a specific syntax, known as rulesets. This is composed of the following:
- Selector - the HTML element name at the start of the ruleset which defines the element(s) to be styled
- Declaration - a single rule like
color: red;
. It specifies which of the element's properties you want to style. - Properties - the attribute of the element you want to style. These include properties such as color, background-color, font-size, etc.
- Property value - the value you apply to the property. This appears to right of the property — after the colon. Depending on the property, you can give it specific values.
From Mozilla's developer docs:
Apart from the selector, each ruleset must be wrapped in curly braces ({}). Within each declaration, you must use a colon (:) to separate the property from its value or values. Within each ruleset, you must use a semicolon (;) to separate each declaration from the next one.
There are many CSS properties you can style. Check out the full list of CSS Properties here.
Adjust the background of your webpage by adding the following code into your style.css
file:
body {
background-color: pink;
}
and change the property value to find something you like. You can use RGB, RGBA, hex, or CSS color values for background-color.
The Box Model
HTML elements are all made up of boxes, and can be thought of as boxes sitting on top of or inside other boxes. A fundamental part of CSS is understanding how to space these boxes, and the box model. Each "box", or HTML element, consists of the following:
- padding, the space around the content. In the example below, it is the space around the paragraph text.
- border, the solid line that is just outside the padding.
- margin, the space around the outside of the border.
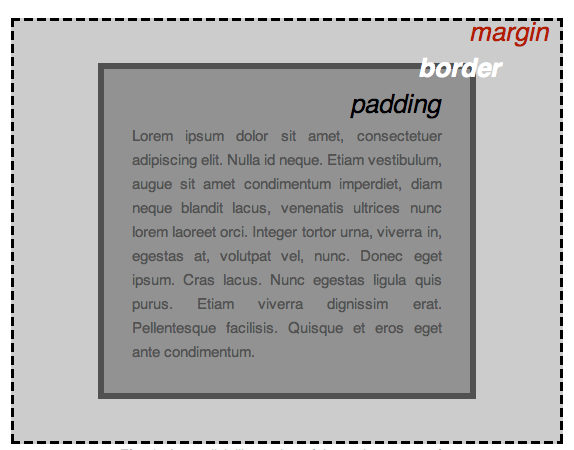
There's a lot more to get into here, but for now try uncommenting the code below this comment in your index.html file
:
<!-- uncomment the code below to display
the right column and local image -->
and the code below this comment in your style.css
file:
/* uncomment the code below to set the grid */
to create a 2 column grid in your webpage
Next Steps
This is the very basics of CSS. To delve further visit Mozilla's CSS Basics webpage (heavily referenced here), and try the Codecademy: Learn CSS module.
Try uncommenting the javascript links:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script> <script src="assets/init.js"></script>
In your HTML file and experimenting with Javascript.
This example uses jquery, which detects "actions" performed on "HTML elements" to perform functions. For instance, this code:
$('body').click(function(){ $(this).css('background', 'yellow'); })
uses the special JQuery selector $
to find the body element, and on click
executes the code between the {}
to change it's background color. Experiment by uncommenting different code blocks and seeing how you can adjust your pages appearance.
You can read more about JQuery here. JQuery isn't the industry standard now, but for personal work and prototyping I think it's very handy.